Creating Animations and Videos with Code: Remotion vs. Motion Canvas
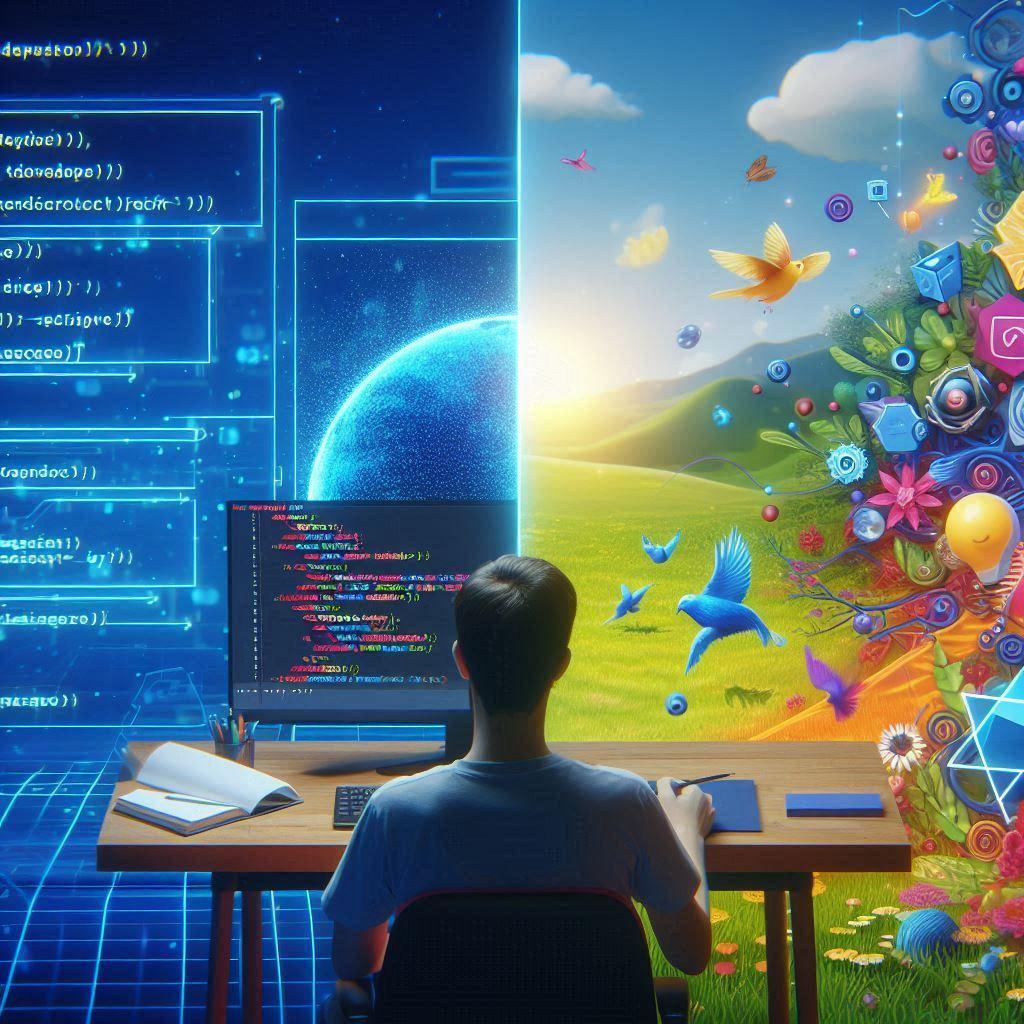
Table Of Content
By CamelEdge
Updated on Wed Nov 20 2024
Introduction
Video and Animation has become an essential medium for storytelling, presentations, and creative expression. Tools like Remotion and Motion Canvas enable developers to create visually engaging animations using code, but they cater to different needs and workflows. In this blog post, we’ll dive deep into what these tools offer, how they differ, and how to use them through practical examples.
What is Remotion?
Remotion is a React-based library for creating animations and videos programmatically. It transforms React components into rendered video files, allowing developers to combine the power of React with tools like CSS, SVG, and JavaScript to create professional-grade videos.
Key Features of Remotion
- React-Based Workflow:
- Remotion uses the familiar React component structure, making it ideal for developers already working with React.
- Media Support:
- Built-in components like
<Video>
,<Audio>
, and<Img>
make it easy to incorporate media into your projects.
- Built-in components like
- Export to Video:
- Render your animations directly as MP4 or other video formats.
- Time-Based Animations:
- Use the timeline to manage animations frame-by-frame.
What is Motion Canvas?
Motion Canvas is a library for creating real-time animations using TypeScript. It’s tailored for interactive animations, making it excellent for presentations, educational content, and real-time visualizations. Unlike Remotion, Motion Canvas focuses more on real-time rendering than video output.
Key Features of Motion Canvas
- Real-Time Animations:
- Motion Canvas excels at producing animations rendered live in the browser or desktop.
- Precision and Control:
- Developers can control every frame of the animation programmatically.
- Interactive Animations:
- Ideal for scenarios requiring user interactions or live updates.
- Audio Synchronization:
- Provides tools for syncing audio with animations.
When to Use Remotion vs. Motion Canvas
Feature | Remotion | Motion Canvas |
---|---|---|
Primary Use Case | Video creation and rendering | Interactive animations |
Image Support | ✅ Built-in | ✅ Built-in |
Video Clip Support | ✅ Built-in <Video> component | ❌ Limited |
Audio Support | ✅ Built-in <Audio> component | ✅ Requires manual integration |
Text Overlays | ✅ Easy to add | ✅ Easy to add |
Transitions | ✅ Predefined or custom | ✅ Requires manual tweening |
Video Export | ✅ Direct video rendering | ❌ Not natively supported |
Interactive Animations | ❌ Not interactive | ✅ Excellent for live updates |
Ease of Use | ✅ Excellent for videos | ✅ Excellent for interactivity |
Getting Started with Remotion
Let’s create a simple video with Remotion that combines images, video clips, audio, text overlays, and transitions.
Installation
Install Remotion in your project:
npx create-video my-video
cd my-video
npm start
This sets up a basic Remotion project.
Code Example: Combining Images, Videos, and Audio
import { Composition, Sequence, Img, Video, Audio, useCurrentFrame, interpolate } from 'remotion';
export const MyComposition: React.FC = () => {
const frame = useCurrentFrame();
return (
<div style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
{/* Image Section */}
<Sequence from={0} durationInFrames={90}>
<Img src="image1.jpg" style={{ opacity: interpolate(frame, [0, 30], [0, 1]) }} />
</Sequence>
{/* Video Section */}
<Sequence from={90} durationInFrames={150}>
<Video src="video1.mp4" />
</Sequence>
{/* Overlay Text */}
<Sequence from={120} durationInFrames={60}>
<h1 style={{ position: 'absolute', top: '50%', left: '50%', transform: 'translate(-50%, -50%)' }}>
Your Text Here
</h1>
</Sequence>
{/* Background Music */}
<Audio src="background-music.mp3" />
</div>
);
};
How It Works
- Images and Videos: Use
<Img>
and<Video>
components to display media. - Transitions: Use
Sequence
andinterpolate
to manage transitions. - Audio: Add background music or narration with the
<Audio>
component.
Rendering the Video
Run the following command to render the video:
npx remotion render src/MyComposition.tsx out/video.mp4
Getting Started with Motion Canvas
Motion Canvas focuses on real-time animations, making it ideal for interactive or presentation-driven animations.
Installation
Add Motion Canvas to your project:
npm install @motion-canvas/core @motion-canvas/2d
Code Example: Adding Audio and Animations
Here’s a simple example where a circle grows in size, synced with audio playback.
import { makeScene2D } from '@motion-canvas/2d';
import { Circle } from '@motion-canvas/2d/lib/components';
import { createRef } from '@motion-canvas/core/lib/utils';
import { AudioPlayer } from '@motion-canvas/core/lib/audio';
import { tween } from '@motion-canvas/core/lib/tweening';
export const SceneWithAudio = makeScene2D(function* (view) {
const circleRef = createRef<Circle>();
view.add(
<Circle
ref={circleRef}
width={100}
height={100}
fill="blue"
/>
);
const audio = new AudioPlayer('path-to-audio-file.mp3');
audio.play();
yield* tween(5, (progress) => {
circleRef().width(100 + progress * 300);
circleRef().height(100 + progress * 300);
});
yield* audio.finished();
});
How It Works
- Audio Sync: Use
AudioPlayer
to synchronize animations with audio. - Animation Control: Define animation timelines using
tween
. - Component Refs: Dynamically update properties of components using
createRef
.
Which One Should You Use?
Choose Remotion If:
- You need to produce a video file.
- Your project involves video clips, audio tracks, and transitions.
- You’re comfortable with React and need a video-focused tool.
Choose Motion Canvas If:
- You’re creating real-time animations for presentations or live interactions.
- Interactivity is a key part of your project.
- You need fine-grained control over animation timelines.
Conclusion
Both Remotion and Motion Canvas are powerful tools, but they cater to distinct use cases. Remotion is the go-to choice for creating polished videos with multiple media types, while Motion Canvas shines in interactive and real-time animation scenarios. By understanding their strengths and limitations, you can select the right tool to bring your creative vision to life.