Secure API with OAuth2, JWT, and AWS Cognito - Part 3
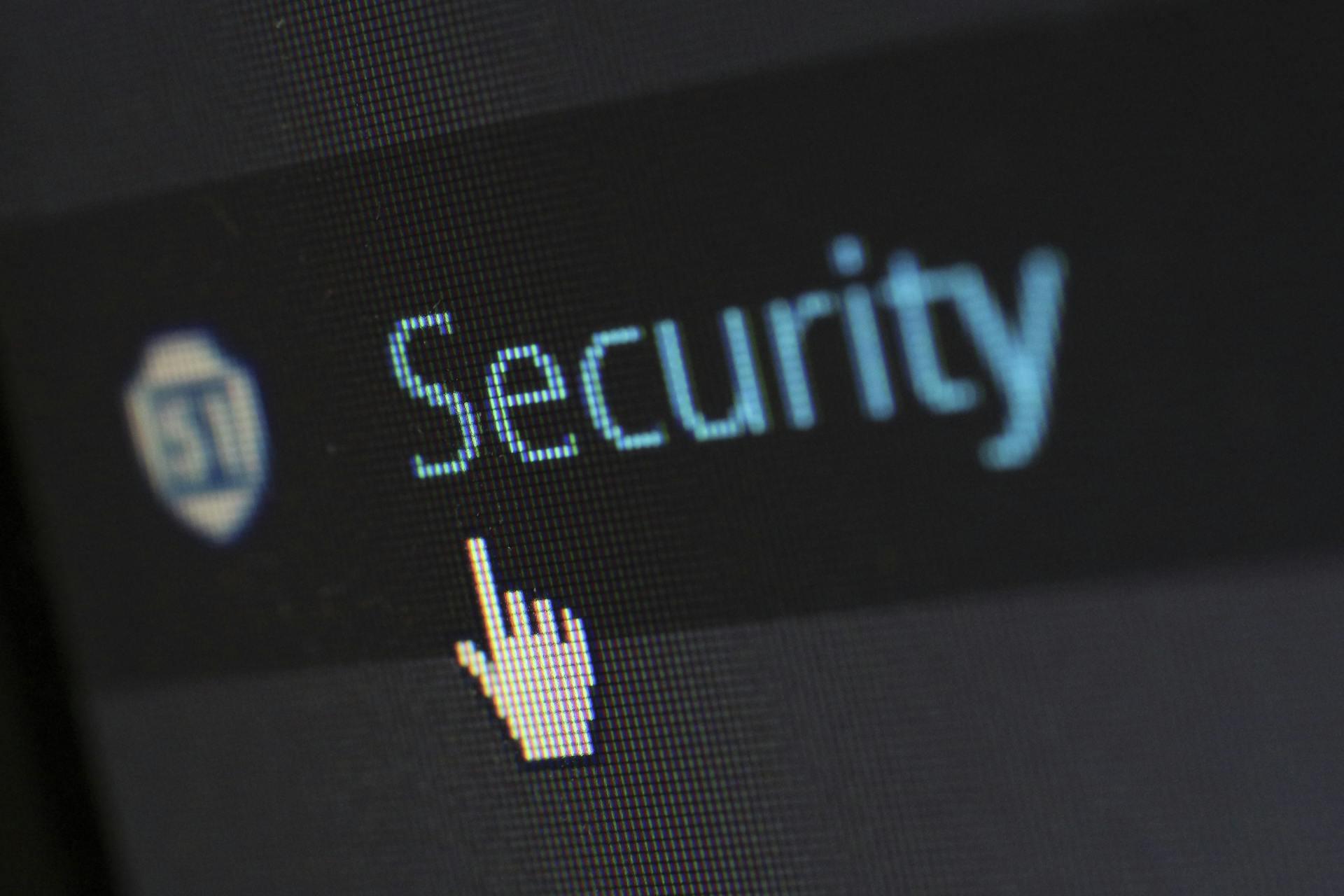
Table Of Content
By CamelEdge
Updated on Mon Jan 20 2025
Introduction
In modern web applications, managing user authentication and authorization is a critical aspect of security and user experience. This implementation demonstrates a robust approach to user authentication by leveraging AWS Cognito for user management and FastAPI for creating APIs. We define models using Pydantic for data validation, ensuring clean and consistent data handling. AWS Cognito provides a scalable and secure solution for handling user signup, verification, signin, token management, and logout.
In part 1, we manually created the JWT token and verified it. In part 2, we use AWS cognito to generate the JWT token which were verified in the FastAPI application. In this part, we will deploy the FastAPI application on AWS Lambda to serve as an authentication service.
AWS Lambda
AWS Lambda is a serverless computing service that allows you to run code without provisioning or managing servers. It automatically scales based on the incoming traffic and charges you only for the compute time consumed. We will deploy the FastAPI application on AWS Lambda using AWS API Gateway to expose the Lambda function as an HTTP endpoint.
We will use AWS CDK to define the infrastructure as code. AWS CDK is an open-source software development framework to define cloud infrastructure in code and provision it through AWS CloudFormation. We will define the Lambda function, API Gateway, and IAM roles using Python code.
Step 1: Install AWS CDK
Start withi installing the AWS CDK CLI using the following link: AWS CDK Installation
Step 2: Create a new AWS CDK project
To create a new AWS CDK project, first create a new directory and navigate into it. Then, run the following command to initialize the project:
mdkir <project-name> && cd <project-name>
cdk init sample-app --language python
This command will create a new AWS CDK project with a sample application in Python. The project structure will look like this:
.
├── app.py
├── cdk.json
├── requirements.txt
├── cdk.out/
├── .env
|── .venv
├── .gitignore
├── README.md
└── cdk/
├── __init__.py
├── cdk_stack.py
Activate the virtual environment and install the required dependencies:
source .venv/bin/activate
python -m pip install -r requirements.txt
Step 3: Define the Lambda function constaining FastAPI application
Create a new folder lambda_auth
and place all your FastAPI application code in this folder. The main application file is called ./lambda_auth/main.py
and a sample file is shown below, where Magnum
is used to wrap the FastAPI application:
from fastapi import FastAPI, Depends
from mangum import Mangum
app = FastAPI(
title="Auth Service",
description="API authentication service",
version="1.0.0",
)
@app.get('/')
def index():
return {"message": "Authentication service"}
handler = Mangum(app)
Step 4: Define the Lambda Layer to include dependencies
The FastAPI application uses libraries that are not available in the AWS Lambda runtime environment. To resolve this, we will use the lambda-layer
to package the dependencies and include them in the Lambda function. Create a new folder layer_auth
and place the following files in it:
.
├── requirements.txt
├── python/
The requirements.txt
file contains the dependencies required by the FastAPI application. These are the dependencies that are not available in the AWS Lambda runtime environment. These are:
boto3
botocore
fastapi
uvicorn
mangum
pydantic
PyJWT
email_validator
cryptography
requests
pydantic-settings
pydantic_core
Install the dependencies using the following command:
python -m pip install -r requirements.txt --platform manylinux2014_x86_64 --target ./python --python-version 3.10 --only-binary=:all:`
This command will install all the dependencies in the python folder. The flag --platform manylinux2014_x86_64
specifies the platform to install the dependencies as the AWS Lambda runtime environment is based on Amazon Linux.
Step 5: Define the Lambda function and API Gateway
Next update the file ./cdk/cdk_stack.py
and define the Lambda function, API Gateway, using the following code:
import os
from constructs import Construct
from aws_cdk import (
Stack,
aws_lambda as _lambda,
aws_apigateway as apigw,
SecretValue as sv,
aws_stepfunctions as sfn
)
from dotenv import load_dotenv
load_dotenv()
class CDKStack(Stack):
def __init__(self, scope: Construct, construct_id: str, **kwargs) -> None:
super().__init__(scope, construct_id, **kwargs)
layer1 = _lambda.LayerVersion(
self, 'layer_auth',
code = _lambda.Code.from_asset('layer_auth'),
compatible_runtimes = [_lambda.Runtime.PYTHON_3_10]
)
# Defines an AWS Lambda resource
my_lambda = _lambda.Function(
self, 'auth',
function_name='AuthFunction',
runtime=_lambda.Runtime.PYTHON_3_10,
code=_lambda.Code.from_asset('lambda_auth'),
handler='main.handler',
layers=[layer1],
)
my_lambda.add_environment('AWS_REGION_NAME', os.environ['AWS_REGION_NAME'])
my_lambda.add_environment('AWS_COGNITO_APP_CLIENT_ID', os.environ['AWS_COGNITO_APP_CLIENT_ID'])
my_lambda.add_environment('AWS_COGNITO_USER_POOL_ID', os.environ['AWS_COGNITO_USER_POOL_ID'])
my_api = apigw.LambdaRestApi(self, "AuthEndpoint", handler=my_lambda)
The above code will create a Lambda function and API Gateway. The Lambda function uses the layer_auth
to include the dependencies required by the FastAPI application. The environment variables are passed to the Lambda function using the add_environment
method. The API Gateway is created using the LambdaRestApi
construct and the Lambda function is passed as the handler.
Step 6: Deploy the application
To deploy the application, run the following commands:
cdk synth
cdk bootstrap
cdk deploy
The cdk synth
command will synthesize the CloudFormation template. The cdk bootstrap
command will create the S3 bucket required by the CDK toolkit. The cdk deploy
command will deploy the application to AWS.
To test the api, go to the AWS console and navigate to the AWS Lambda service. Click on the Lambda function and copy the API Gateway URL. You can test the API using the URL in the browser or using a tool like Postman.
Conclusion
In this part, we deployed the FastAPI application on AWS Lambda using AWS CDK. We used the lambda-layer
to package the dependencies required by the FastAPI application. The Lambda function was created using the Function
construct and the API Gateway was created using the LambdaRestApi
construct.
Related