Understanding Blob Detection: Multiscale Space Filtering and Its Importance
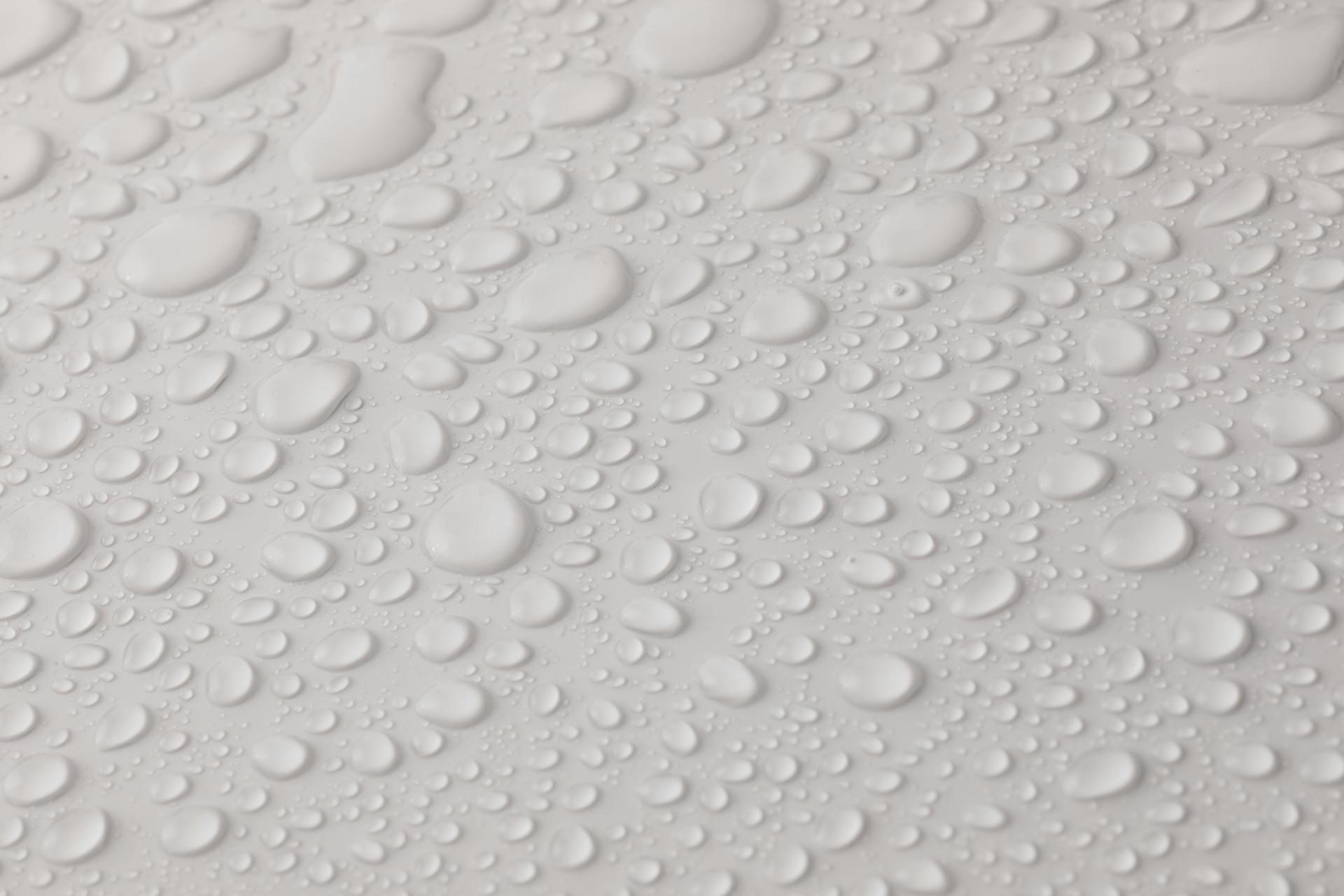
Table Of Content
By CamelEdge
Updated on Tue Aug 06 2024
Introduction
In the field of computer vision, detecting features within images is a fundamental task. Among various features, "blobs" are particularly important as they represent regions of interest that differ in properties like brightness or texture from their surroundings. Blob detection techniques are employed to identify and analyze these regions, allowing for applications such as object recognition, image segmentation, and feature matching.
One key concept in effective blob detection is multiscale space filtering. This approach involves applying filters at multiple scales or levels of detail, which enables the detection of features that may vary in size or resolution. Understanding this concept is crucial for building robust computer vision systems that can operate effectively across a wide range of image conditions.
Before diving into multiscale space filtering, it's important to understand what we mean by a blob
. In image processing, a blob is essentially a region in an image where some properties, such as intensity or texture, are uniform or vary smoothly. Blobs can be thought of as areas that are visually distinct from their surroundings, and detecting these regions is vital for many computer vision applications.
The Challenge of Scale in Feature Detection
One of the major challenges in detecting features like blobs is handling the scale at which these features appear. Different objects or structures in an image can exist at various sizes, and detecting them accurately requires a method that can adapt to these scale differences.
A classic example of this challenge is seen in corner detection using the Harris Corner Detector. While the Harris Corner Detector is effective at identifying corners, it is not scale-invariant. This means that if the size of a corner changes due to scaling (e.g., when an object is closer or farther away), the detector might fail to identify it correctly. This limitation arises because the same window size is used to detect corners, regardless of the scale at which they appear. To detect larger corners, we would need larger windows, which complicates the detection process.
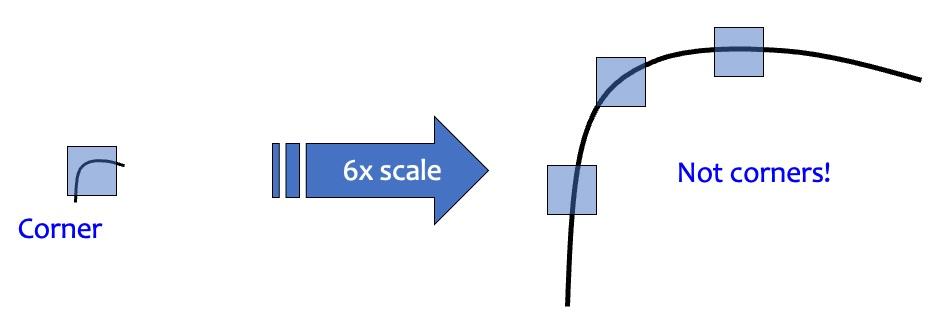
The Solution: Multiscale Space Filtering
To address the challenge of scale, the concept of multiscale space filtering is employed. This method involves applying filters at different scales, allowing for the detection of features at various levels of detail within an image. By examining an image across multiple scales, we can identify blobs that might otherwise be missed if we only analyzed the image at a single scale.
What is a Blob?
A "blob" is a region of an image that stands out from its surroundings due to consistent intensity or texture. But, more technically, a blob can be understood as a superposition of edges in one dimension (1D).
To visualize this, imagine an edge in a 1D signal, like a sharp transition from dark to light. If you combine this with another edge that transitions from light to dark, you form a "blob" a distinct region where the intensity or texture changes significantly compared to the surrounding area. This blob represents a feature or an area of interest that we want to detect in an image.
Blob Detection using a filter
To detect a blob in an image, we use a technique called convolution. Convolution involves sliding a filter, which can be thought of as a small "picture" of the feature we are trying to detect, across the entire image. At each position, the filter compares its pattern to the local region of the image, highlighting areas where the match is strong.
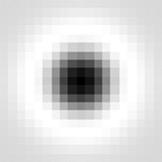
For detecting blobs, the filter we use should resemble the shape of a blob at the specific scale we are interested in. This filter is known as the Laplacian of Gaussian (LoG). The LoG filter is particularly effective for blob detection because it is designed to respond strongly to circular regions in the image where intensity changes sharply.
By adjusting the radius of the LoG filter (scale), we can detect blobs of different sizes, making this method adaptable to various scales within the image. This ability to scale the filter is crucial for accurately identifying blobs, regardless of their size or the resolution of the image.
Laplacian of Gaussian
The Laplacian of Gaussian (LoG) is a popular filter blob detection. It combines two operations: Gaussian smoothing and the Laplacian operator, which is the second derivative that measures the rate at which the gradient changes.
The LoG filter is mathematically represented as:
Where:
- are the coordinates of the point in the filter.
- is the standard deviation of the Gaussian distribution, controlling the scale of the smoothing.
- The factor ensures that the filter is properly normalized, so the output is appropriately scaled.
The LoG function is essentially the Laplacian (second derivative) of the Gaussian function.
Implementation
There are two way the LoG filter can be implemented.
- After computing the second derivative of the Gaussian filter, , we then convolve this with the image. This operation can be represented as , where the Laplacian of Gaussian filter is applied to the image through convolution.
def LoG(ksize, sigma):
# returns sigma normalized values
assert ksize%2 == 1
x, y = np.meshgrid(np.arange(-(ksize // 2),
ksize // 2 + 1),
np.arange(-(ksize // 2), ksize // 2 + 1))
return 483*sigma**2 * -1/(np.pi * sigma**4) *
(1 - (x**2 + y**2)/(2*sigma**2)) *
np.exp(- (x**2 + y**2)/(2*sigma**2))
LoG = LoG(15, 3)
response = cv2.filter2D(image, cv2.CV_32F, LoG)
- Another equivalent approach is to first convolve the image with the Gaussian filter, , and then apply the Laplacian operator. Mathematically, this can be expressed as:
Applying the Laplacian after blurring with a Gaussian filter is mathematically equivalent to directly convolving the image with a LoG filter.
image = cv2.imread('<image filenmae>', cv2.IMREAD_GRAYSCALE)
image = cv2.GaussianBlur(image, ksize=(9,9), sigmaX = 3, sigmaY = 3)
# Apply the laplacian
response = cv2.Laplacian(image, cv2.CV_32F, ksize=21, scale=5)
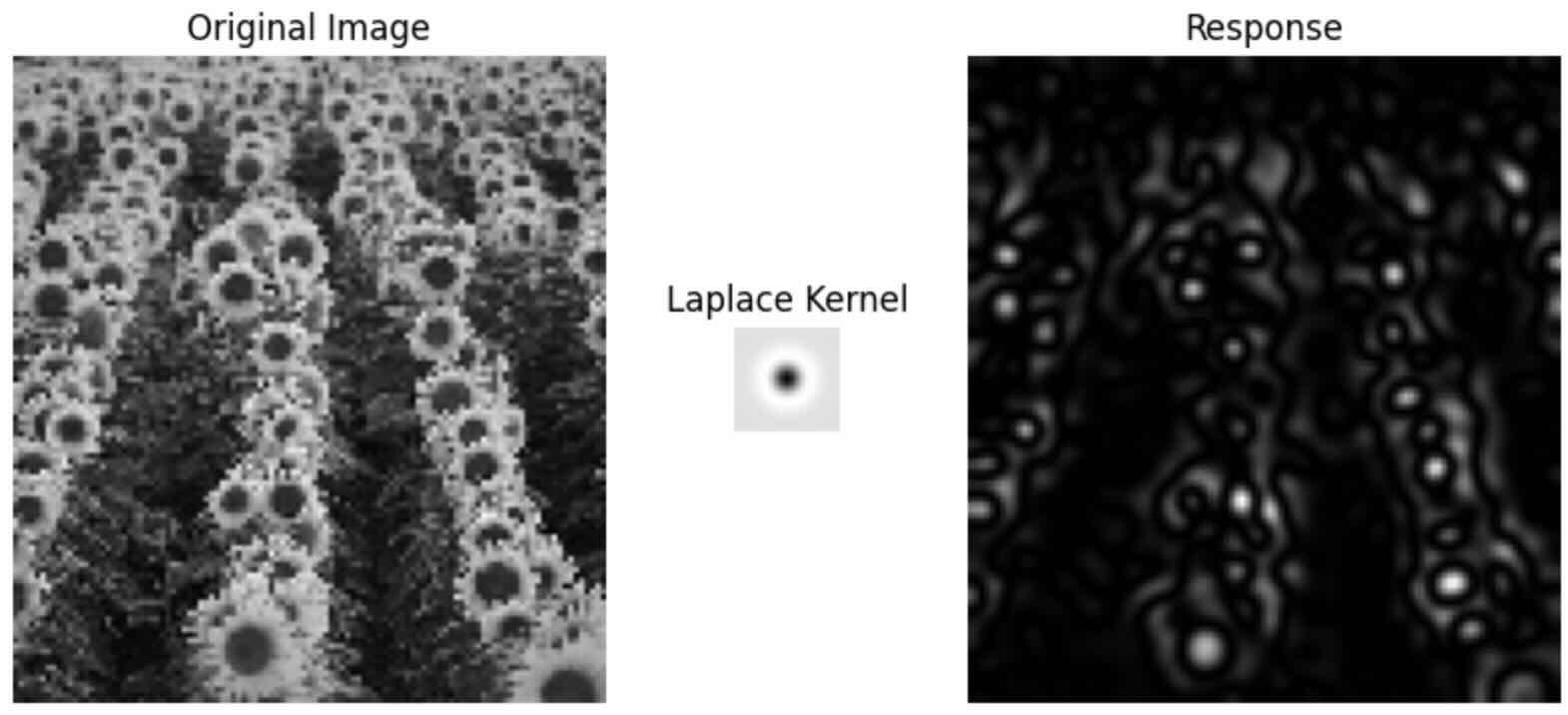
Detecting Blobs at Different Scales
One of the strengths of the LoG filter is its ability to detect blobs at different scales. By adjusting the radius of the filter, we can tune the size of the blobs that we want to detect. A smaller radius will detect smaller blobs, while a larger radius will be sensitive to larger blobs.
This scale adaptability is crucial in image processing because features in images can vary significantly in size. By using a multiscale approach with the LoG filter, we can ensure that blobs of all sizes are detected, providing a comprehensive analysis of the image.
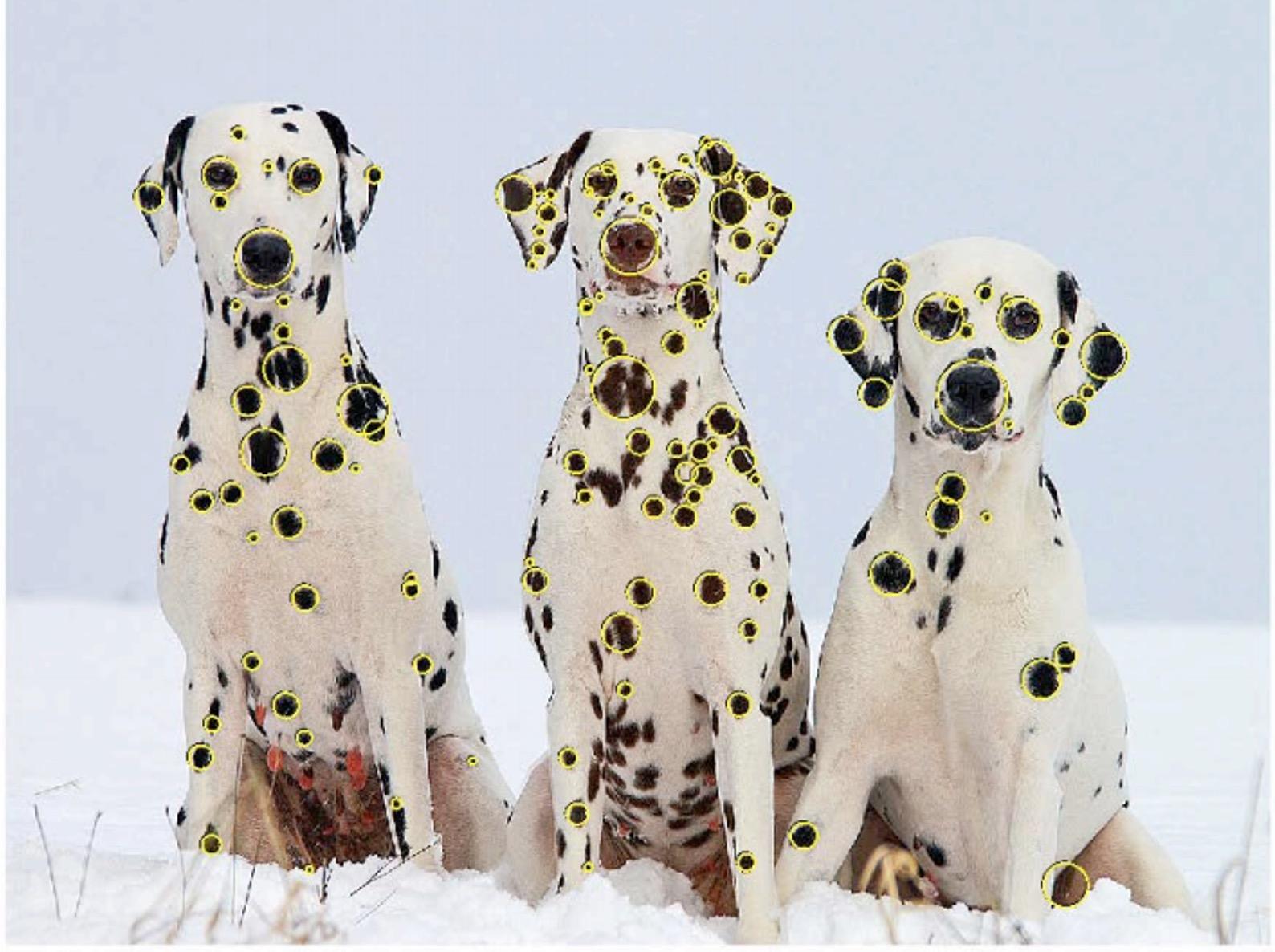
Characteristic Scale of a Blob
The characteristic scale of a blob is the scale at which the blob generates the strongest response. To identify this characteristic scale, we convolve the image with scale-normalized Laplacians at various scales and identify the scale that produces the maximum response.
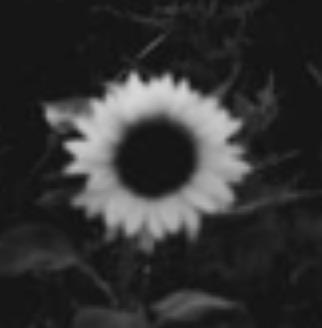
The response of the Laplacian of Gaussian (LoG) filter varies across different scales. The maximum response occurs when the filter size matches the scale of the blob, which is known as the characteristic scale.
How Multiscale Space Filtering Works
-
Scale-Space Representation: The first step in multiscale space filtering is to create a scale-space representation of the image. This is typically done by progressively smoothing the image with a Gaussian filter of increasing standard deviation. Each smoothed version of the image represents the original image at a different scale, where fine details are gradually removed as the scale increases.
-
Feature Detection at Multiple Scales: Once we have a scale-space representation, feature detection (such as blob detection) is performed independently at each scale. This involves applying the Laplacian of Gaussian (LoG) at each scale.
-
Finding Blobs Across Scales: After detecting features at various scales, the results are merged to identify significant blobs that stand out across different levels of detail. This process involves considering the characteristic scale of each blob.
Advantages of Multiscale Space Filtering
-
Scale Invariance: By analyzing an image at multiple scales, the detector becomes less sensitive to the specific size of the features, making it more robust to changes in scale.
-
Enhanced Feature Detection: Multiscale filtering allows for the detection of both small and large features, which might be missed if only a single scale is considered.
Conclusion
Multiscale space filtering is a powerful technique in computer vision that addresses the challenge of scale in feature detection. By applying filters at different scales, this approach allows for the detection of blobs and other features across various levels of detail, ensuring a more comprehensive analysis of images. This technique overcomes the limitations of traditional methods like the Harris Corner Detector, which are not scale-invariant, and it opens the door to more robust and flexible computer vision applications.
Understanding and implementing multiscale space filtering can significantly enhance the performance of computer vision systems, making them more effective in diverse and dynamic environments. Whether you're working on object recognition, image segmentation, or any other feature detection task, incorporating this approach will undoubtedly lead to more reliable and accurate results.
Here are three FAQ entries based on your blog content:
FAQs
- What is a blob in image processing?
A blob in image processing is a distinct region in an image where properties such as intensity or texture are consistent or vary smoothly compared to its surroundings. Essentially, blobs are areas that stand out due to significant changes in image attributes. Detecting blobs is important for tasks like object recognition and image segmentation as these regions often contain crucial information.
- Why is multiscale space filtering important for blob detection?
Multiscale space filtering is crucial for blob detection because it allows for the detection of features across various sizes and levels of detail. By applying filters at different scales, this technique can identify blobs that might be missed if only a single scale is considered. This approach helps address the challenge of scale variance in images, ensuring that both small and large blobs are detected effectively.
- How does the Laplacian of Gaussian (LoG) filter help in detecting blobs?
The Laplacian of Gaussian (LoG) filter is designed to detect blobs by responding strongly to circular regions in an image where intensity changes sharply. It combines Gaussian smoothing with the Laplacian operator to highlight regions that stand out due to rapid changes in image properties. By adjusting the scale of the LoG filter, you can detect blobs of various sizes, making it a versatile tool for blob detection in multiscale space filtering.
Related Posts